Context API – Global State Management
The Context API in React Native provides a way to pass data through the component tree without having to pass props down manually at every level. It's particularly useful for sharing stateful data, such as theme preferences, user authentication status, or language preferences, across multiple components in an application.
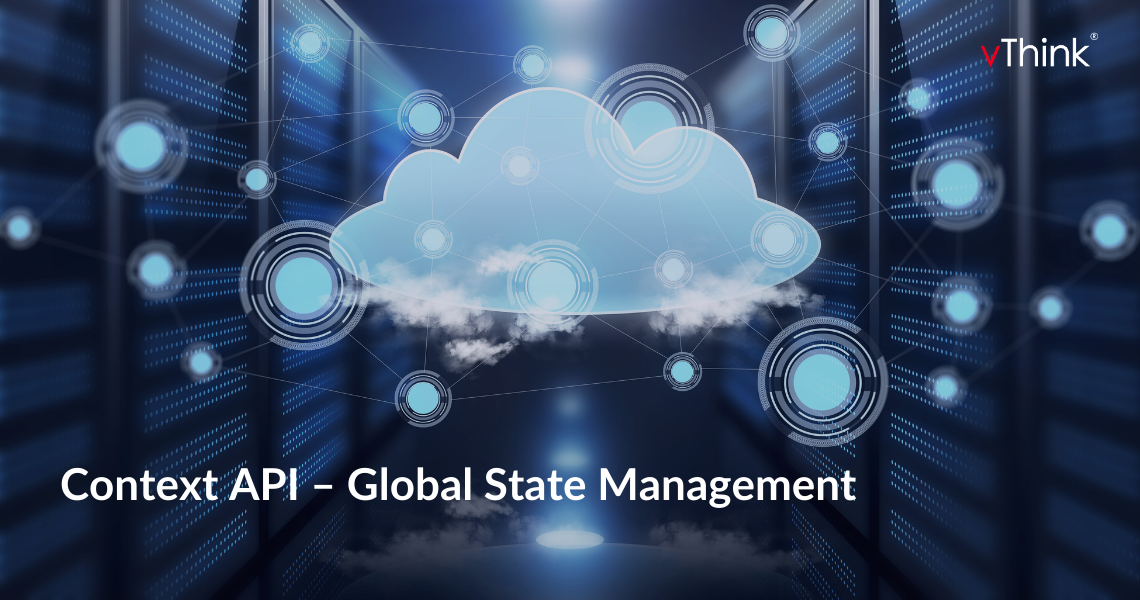
Introduction
The Context API in React Native provides a way to pass data through the component tree without having to pass props down manually at every level. It's particularly useful for sharing stateful data, such as theme preferences, user authentication status, or language preferences, across multiple components in an application.
With the Context API, you can create a "context" object that holds the shared data and provides it to any component within the tree. Components can then access this data using the useContext hook or the Consumer component, making it easy to access and update shared state without prop drilling.
The Context API simplifies state management in React Native applications, especially for complex applications with deeply nested component trees or components that need access to the global state. It promotes cleaner, more modular code by decoupling state management logic from individual components.
When to Use Context
Context is designed to share data that can be considered “global” for a tree of React components, such as the current authenticated user, theme, or preferred language. For example, in the code below we manually thread through a “theme” prop in order to style of the components in an application.
Comparison Between Props Drilling and Context API


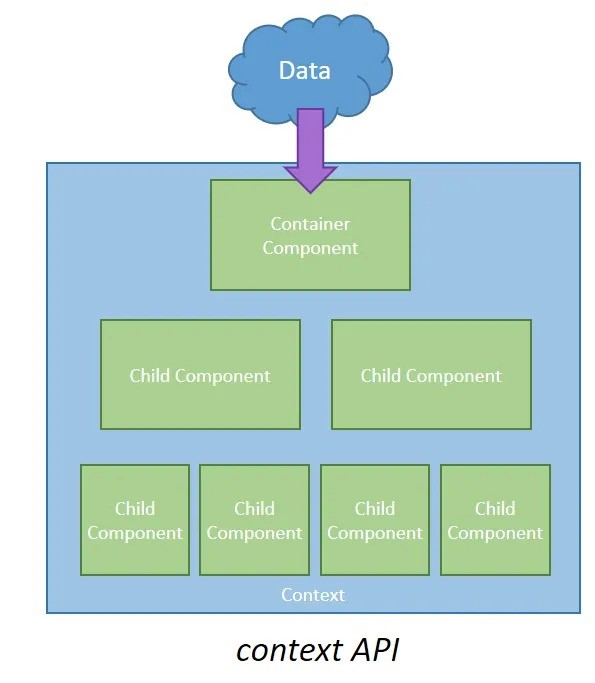
Advantages of Context API
- Global State Management: Context API allows you to manage global state in your React Native application without having to pass props down through every level of your component tree. This simplifies state management, especially for larger applications with complex data flows.
- Avoids Prop Drilling: Context API helps you avoid prop drilling, which is the process of passing props through multiple levels of nested components. This improves code readability and maintainability by reducing the complexity of your component hierarchy.
- Simplifies Component Composition: By providing a centralized location for storing and updating state, the Context API simplifies the process of composing your React Native components. Components can easily access the data they need without having to worry about where that data is coming from.
- Encourages Reusability: Context API encourages reusability by allowing you to create context providers that encapsulate certain pieces of functionality or state. These providers can then be reused across multiple components or even multiple applications, promoting code modularity and scalability.
- Performance Optimization: Context API can help optimize performance by reducing the number of unnecessary re-renders in your application. Since components only re-render when their relevant context values change, you can avoid unnecessary renders caused by changes in unrelated parts of your component tree.
- Easier Testing: With Context API, you can separate concerns more effectively, making it easier to test individual components in isolation. This improves the overall testability of your React Native application and facilitates the adoption of test-driven development practices.
- Integration with Third-party Libraries: Many third-party libraries and tools in the React Native ecosystem support integration with Context API, making it easier to incorporate additional functionality into your application while maintaining a consistent state management approach.
Overall, the Context API in React Native provides a flexible and efficient way to manage state and share data between components, leading to cleaner code, improved performance, and enhanced developer productivity.
Disadvantages of Context API
- Overuse Can Lead to Performance Issues: Using a single context for multiple unrelated states can cause unnecessary re-renders.
- Not Suitable for Complex State Management: For applications with highly dynamic and complex state requirements, solutions like Redux or MobX might be more suitable.
Real-Time Implementation Example
Here, uses context API for theme change.
Steps
1. Create a Theme Context:
First, you create a Context object to hold information about the current theme, including properties like background color, text color, etc.

2. Create a Theme Provider:
Next, you create a Provider component that wraps your entire application. This Provider component will manage the current theme state and provide methods to update the theme.
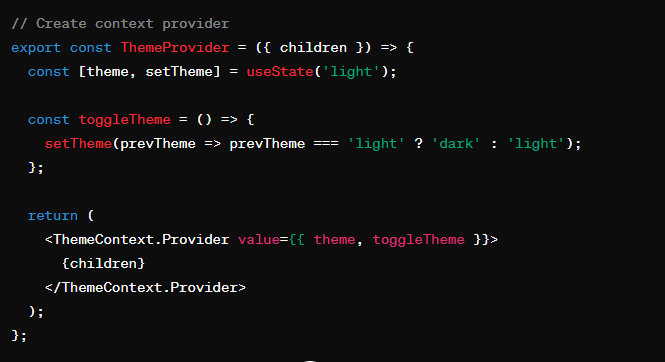
3. Wrap your App with the Theme Provider:
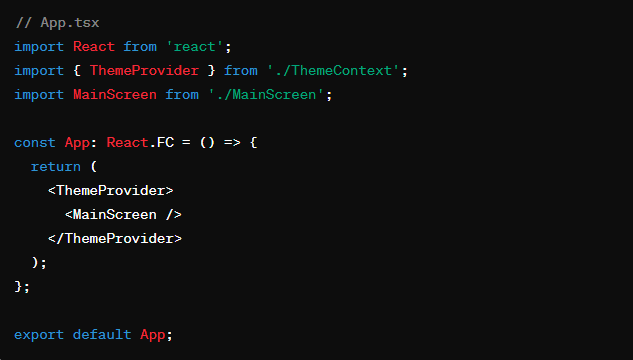
4. Use the Theme Context in Components:
Components throughout your application can access the current theme using the useContext hook provided by React. This allows them to dynamically style their content based on the current theme.

Update Theme Dynamically:
When a user wants to switch the theme, simply update the theme state in the Provider component. This change will automatically propagate to all components that are consuming the theme context, causing them to re-render with the new theme.
Conclusion
The Context API in React offers a straightforward way to manage the global state, allowing components to access shared data without prop drilling. It's efficient, easy to use, and helps maintain a clean and organized codebase by centralizing state management.