Advanced Query Optimization Techniques in Multi-Tenant Databases
Multi-tenant databases are a common architectural choice for SaaS applications, where multiple customers (tenants) share the same database while maintaining data isolation. This approach offers cost-efficiency and simplified management but introduces unique challenges, especially around query performance and resource contention. Optimizing queries in a multi-tenant database is critical to ensure scalability, reliability, and performance.
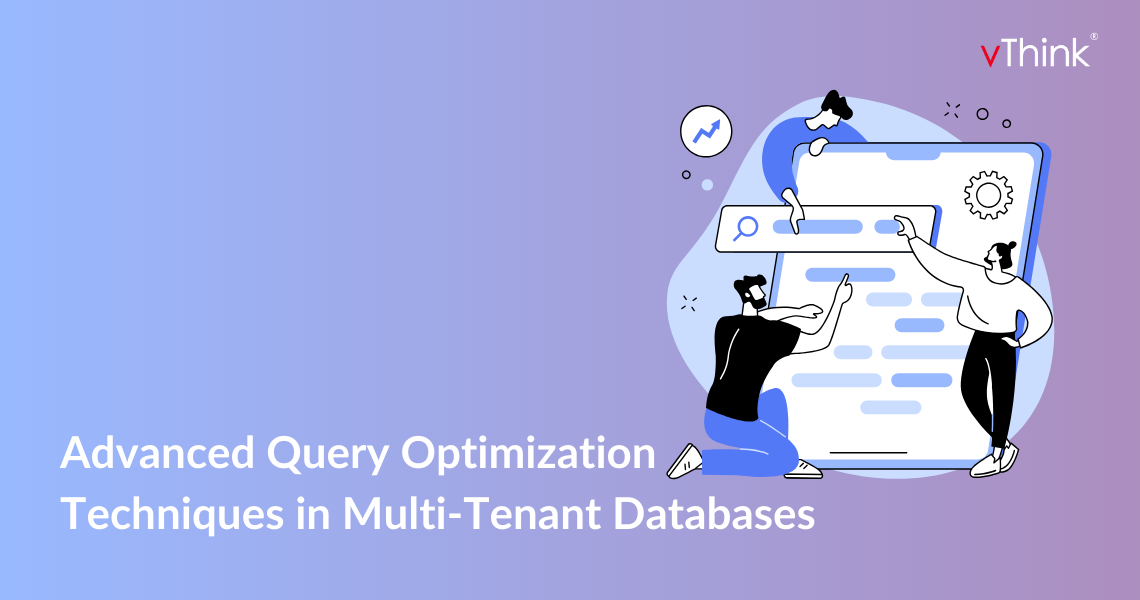
Introduction
Multi-tenant databases are a common architectural choice for SaaS applications, where multiple customers (tenants) share the same database while maintaining data isolation. This approach offers cost-efficiency and simplified management but introduces unique challenges, especially around query performance and resource contention. Optimizing queries in a multi-tenant database is critical to ensure scalability, reliability, and performance.
Here, we’ll explore advanced query optimization techniques tailored for multi-tenant databases, with a focus on practical implementations in relational databases like PostgreSQL, MySQL, and MSSQL.
Understanding Multi-Tenant Architecture
In a multi-tenant database, tenants share the same schema, and tenant-specific data is typically isolated using a tenant ID. This design often results in:
- High data volume and table bloat as tenants grow.
- Diverse query patterns across tenants.
- Resource contention between tenants.
Query optimization in this setup ensures that:
- Tenant-specific queries execute efficiently.
- Resource usage remains balanced across tenants.
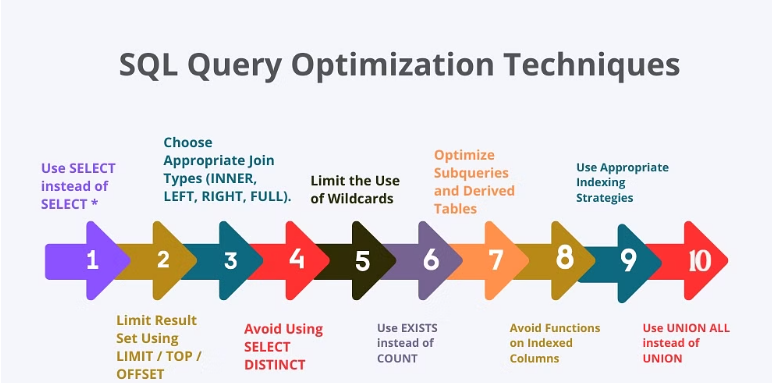
Challenges in Query Optimization for Multi-Tenant Databases
- High Query Complexity: Queries often include filters for tenant isolation, leading to large execution plans.
- Uneven Data Distribution: Some tenants may have significantly more data than others, causing query performance issues.
- Resource Contention: Multiple tenants querying the database simultaneously can lead to I/O, CPU, and memory contention.
- Index Maintenance: Frequent writes from multiple tenants can cause index fragmentation.
Advanced Query Optimization Techniques
1. Partitioning for Tenant Isolation
Partitioning divides a table into smaller, more manageable pieces, improving query performance by limiting the amount of data scanned.
- PostgreSQL: Use table partitioning by tenant ID.
CREATE TABLE sales (
id SERIAL PRIMARY KEY,
tenant_id INT NOT NULL,
sale_date DATE NOT NULL,
amount NUMERIC
) PARTITION BY LIST (tenant_id);
- Benefits:
- Query performance improves as only relevant partitions are scanned.
- Easier to manage large datasets.
2. Query Hints for Optimized Plans
Modern RDBMSs allow the use of query hints to guide the optimizer.
- SQLServer:
Force specific indexes or join strategies for better performance.
SELECT *
FROM orders WITH (INDEX(idx_tenant_date))
WHERE tenant_id = 101 AND order_date > '2024-01-01';
- MySQL:
Use optimizer hints like USE INDEX or STRAIGHT_JOIN.
SELECT * FROM orders USE INDEX (idx_tenant_id) WHERE tenant_id = 101;
3. Tenant-Specific Indexing
Custom indexes for high-frequency queries can significantly improve performance.
- For tenants with skewed data, consider creating partial indexes:
- PostgreSQL:
CREATE INDEX idx_tenant_orders ON orders (order_date) WHERE tenant_id = 101;
- Reduces index size and speeds up queries for specific tenants.
4. Query Plan Caching
Query execution plans can vary by tenant due to differences in data volume. Plan caching ensures consistent performance for frequently executed queries.
- SQL Server Query Store: Track and force optimal plans for tenant-specific queries.
EXEC sp_query_store_force_plan @query_id = 123, @plan_id = 456;
- PostgreSQL: Use prepared statements for recurring queries.
PREPARE tenant_query (INT) AS
SELECT * FROM orders WHERE tenant_id = $1 AND order_date > '2024-01-01';
EXECUTE tenant_query(101);
5. Rate Limiting and Throttling
Implement query throttling to prevent resource contention caused by noisy tenants.
- AWS RDS Proxy: Use connection pooling and throttling to manage query concurrency.
- Application Layer: Introduce limits on tenant query execution time or result size.
6. Analyzing Query Plans
Tools like EXPLAIN and EXPLAIN (ANALYZE) help identify bottlenecks in tenant queries.
- PostgreSQL:
EXPLAIN (ANALYZE, BUFFERS)
SELECT * FROM orders WHERE tenant_id = 101;
- Look for:
- Sequential scans on large tables.
- High buffer usage or I/O waits.
- SQL Server: Use Query Performance Insights or Execution Plans in SSMS to identify expensive operations.
7. Leveraging Multi-Tenant Extensions in Cloud
- AWS Aurora:
Use Aurora’s query caching and read replicas for tenant query segregation.
- PostgreSQL: Leverage extensions like pg_partman for automated partition management.
Optimizing Multi-Tenant Databases in AWS
AWS offers a range of features to optimize multi-tenant database performance, specifically for RDS, Aurora, and PostgreSQL environments.
1. AWS RDS Optimizations
AWS RDS provides several optimization features that can be leveraged to enhance the performance of multi-tenant databases:
- RDS Instance Types and Scaling: Choose the appropriate instance type for the database workload, and scale horizontally with read replicas to offload read-intensive queries.
- RDS Proxy: By pooling database connections and enabling automatic failover, RDS Proxy can help manage concurrency and improve overall performance during peak loads.
- Monitoring Tools: Use Amazon CloudWatch and Performance Insights to monitor database performance metrics such as CPU usage, memory utilization, and I/O activity, which are critical in identifying and mitigating resource contention.
2. Aurora for Multi-Tenant Database Optimization
Amazon Aurora, with its unique architecture, provides several benefits for multi-tenant databases:
- Aurora’s Query Caching: Helps reduce the time for query execution by caching frequently executed queries.
- Aurora Read Replicas: Distribute read-heavy tenant queries to read replicas, reducing the load on the primary instance and improving performance.
3. PostgreSQL on AWS
- Extensions: Use PostgreSQL extensions like pg_partman for partition management, pg_stat_statements for query analysis, and pg_repack for online index reorganization.
- Performance Insights: Amazon RDS Performance Insights helps identify bottlenecks, track query performance over time, and drill down into the most resource-intensive queries.
Case Study: Optimizing Multi-Tenant Queries in AWS
Scenario:
A SaaS platform using AWS RDS for PostgreSQL experienced slow query performance for large tenants during peak hours.
Solution:
- Partitioning: Implemented list partitioning by tenant ID for the largest tables.
- Query Optimization: Added partial indexes for high-frequency tenant queries.
- Read Replicas: Redirected read-intensive tenant queries to read replicas using AWS RDS Proxy.
- Resource Throttling: Enforced query concurrency limits using application-level rate limiting.
Result:
- Query response times improved by 50%.
- Resource contention during peak hours reduced by 40%.
Best Practices for Query Optimization in Multi-Tenant Databases
- Monitor Regularly: Use tools like AWS CloudWatch, Performance Insights, or pg_stat_statements for query performance monitoring.
- Optimize for Hot Tenants: Focus on tenants with high data volumes or query frequency.
- Scale Strategically: Consider sharding or moving large tenants to dedicated instances.
- Test Changes: Validate optimizations in a staging environment to avoid tenant disruptions.
Conclusion
Optimizing queries in a multi-tenant database requires a combination of advanced techniques and strategic resource management. By leveraging tools like partitioning, query plan caching, and resource throttling, DBAs can ensure a consistent and scalable performance for tenants in shared environments. Cloud-native features in platforms like AWS RDS further enhance optimization capabilities, making multi-tenant architectures more efficient and cost-effective.